Commands are used to remove tight coupling. It helps in decoupling the code from the code behind. And hence it offers reusable code that can be called or used multiple times.
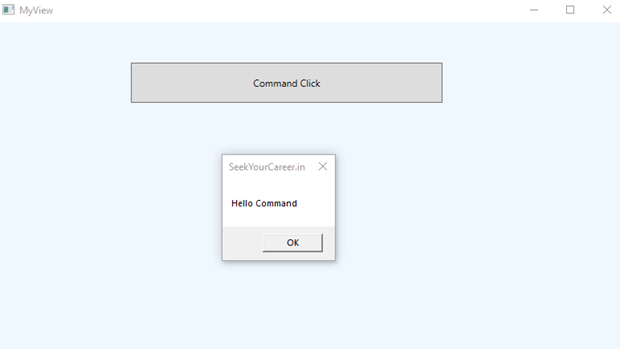

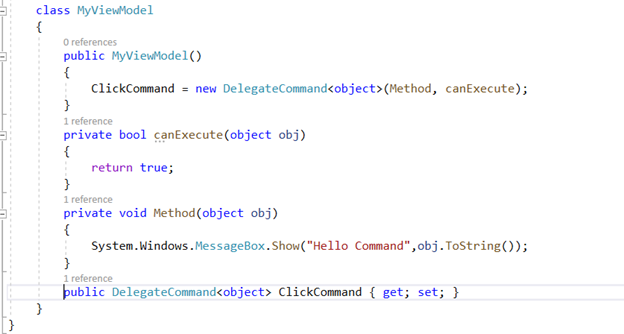
//Create this class one time throughout the application
namespace WPF_Demo
{
public class DelegateCommand<T> : ICommand
{
private Predicate<T> _canExecute;
private Action<T> _method;
bool _canExecuteCache = true;
public DelegateCommand(Action<T> method) : this(method, null)
{
}
public DelegateCommand(Action<T> method, Predicate<T> canExecute)
{
_method = method;
_canExecute = canExecute;
}
public bool CanExecute(object parameter)
{
if (_canExecute != null)
{
bool tempCanExecute = _canExecute((T)parameter);
if (_canExecuteCache != tempCanExecute)
{
_canExecuteCache = tempCanExecute;
this.RaiseCanExecuteChanged();
}
}
return _canExecuteCache;
}
public void RaiseCanExecuteChanged()
{
if (CanExecuteChanged != null)
{
CanExecuteChanged(this, new EventArgs());
}
}
public void Execute(object parameter)
{
if (_method != null)
_method.Invoke((T)parameter);
}
#region ICommand Members
public event EventHandler CanExecuteChanged;
#endregion
}
}
VIEWMODEL.CS
namespace WPF_Demo.ViewModel
{
class MyViewModel
{
public MyViewModel()
{
ClickCommand = new DelegateCommand<object>(Method, canExecute);
}
private bool canExecute(object obj)
{
return true;
}
private void Method(object obj)
{
System.Windows.MessageBox.Show("Hello Command",obj.ToString());
}
public DelegateCommand<object> ClickCommand { get; set; }
}
}
XAML.CS
<Button Grid.Column="0" Grid.Row="1" Content="Command Click" Command="{Binding ClickCommand}" CommandParameter="100" />